Features of PHP
The main features of php is; it is open source scripting language so you can free download this and use. PHP is a server site scripting language. It is open source scripting language. It is widely used all over the world. It is faster than other scripting language. Some important features of php are given below;
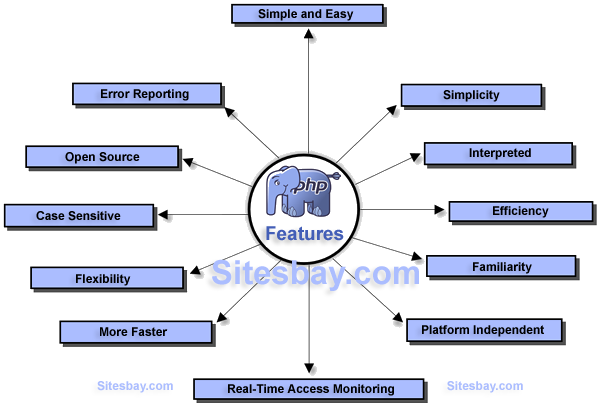
Features of php
It is most popular and frequently used world wide scripting language, the main reason of popularity is; It is open source and very simple.
- Simple
- Faster
- Interpreted
- Open Source
- Case Sensitive
- Simplicity
- Efficiency
- Platform Independent
- Security
- Flexibility
- Familiarity
- Error Reporting
- Loosely Typed Language
- Real-Time Access Monitoring
Simple
It is very simple and easy to use, compare to other scripting language it is very simple and easy, this is widely used all over the world.
Interpreted
It is an interpreted language, i.e. there is no need for compilation.
Faster
It is faster than other scripting language e.g. asp and jsp.
Open Source
Open source means you no need to pay for use php, you can free download and use.
Platform Independent
PHP code will be run on every platform, Linux, Unix, Mac OS X, Windows.
Case Sensitive
PHP is case sensitive scripting language at time of variable declaration. In PHP, all keywords (e.g. if, else, while, echo, etc.), classes, functions, and user-defined functions are NOT case-sensitive.
Error Reporting
PHP have some predefined error reporting constants to generate a warning or error notice.
Real-Time Access Monitoring
PHP provides access logging by creating the summary of recent accesses for the user.
Loosely Typed Language
PHP supports variable usage without declaring its data type. It will be taken at the time of the execution based on the type of data it has on its value.
Connection Using PHP Script
PHP provides mysql_connect() function to open a database connection
Syntax
connection mysql_connect(server,user,passwd,new_link,client_flag);
Create a Database using PHP Script
PHP uses mysql_query function to create or delete a MySQL database.
Syntax
bool mysql_query( sql, connection );
Selecting a MySQL Database Using PHP Script
PHP provides function mysql_select_db to select a database. It returns TRUE on success or FALSE on failure.
Syntax
bool mysql_select_db( db_name, connection );
Deleting Data Using a PHP Script
You can use the SQL DELETE command with or without the WHERE CLAUSE into the PHP function – mysql_query(). This function will execute the SQL command in the same way as it is executed at the mysql>
Syntax
DELETE FROM table_name [WHERE Clause]
PHP Functions
PHP function is a piece of code that can be reused many times. It can take input as argument list and return value. There are thousands of built-in functions in PHP.
Advantage of PHP Functions
Code Reusability: PHP functions are defined only once and can be invoked many times, like in other programming languages.
Less Code: It saves a lot of code because you don’t need to write the logic many times. By the use of function, you can write the logic only once and reuse it.
Easy to understand: PHP functions separate the programming logic. So it is easier to understand the flow of the application because every logic is divided in the form of functions.
PHP User-defined Functions
We can declare and call user-defined functions easily. Let’s see the syntax to declare user-defined functions.
Syntax
- function functionname(){
- //code to be executed
- }
PHP Functions Example
File: function1.php
- <?php
- function sayHello(){
- echo “Hello PHP Function”;
- }
- sayHello();//calling function
- ?>
Output:
PHP Function Arguments
We can pass the information in PHP function through arguments which is separated by comma.
PHP supports Call by Value (default), Call by Reference, Default argument values and Variable-length argument list.
Let’s see the example to pass single argument in PHP function.
File: functionarg.php
- <?php
- function sayHello($name){
- echo “Hello $name<br/>”;
- }
- sayHello(“Sonoo”);
- sayHello(“Vimal”);
- sayHello(“John”);
- ?>
Output:
Hello Sonoo Hello Vimal Hello John
PHP Call By Reference
Value passed to the function doesn’t modify the actual value by default (call by value). But we can do so by passing value as a reference.
By default, value passed to the function is call by value. To pass value as a reference, you need to use ampersand (&) symbol before the argument name.
Let’s see a simple example of call by reference in PHP.
File: functionref.php
- <?php
- function adder(&$str2)
- {
- $str2 .= ‘Call By Reference’;
- }
- $str = ‘Hello ‘;
- adder($str);
- echo $str;
- ?>
Output:
Hello Call By Reference
PHP What is OOP?
OOP stands for Object-Oriented Programming.
Procedural programming is about writing procedures or functions that perform operations on the data, while object-oriented programming is about creating objects that contain both data and functions.
Object-oriented programming has several advantages over procedural programming:
- OOP is faster and easier to execute
- OOP provides a clear structure for the programs
- OOP helps to keep the PHP code DRY “Don’t Repeat Yourself”, and makes the code easier to maintain, modify and debug
- OOP makes it possible to create full reusable applications with less code and shorter development time
Define a Class
A class is defined by using the class
keyword, followed by the name of the class and a pair of curly braces ({}). All its properties and methods go inside the braces:
Syntax
<?php
class Fruit {
// code goes here…
}
?>
Define Objects
Classes are nothing without objects! We can create multiple objects from a class. Each object has all the properties and methods defined in the class, but they will have different property values.
Objects of a class is created using the new
keyword.
Example
<?php
class Fruit {
// Properties
public $name;
public $color;// Methods
function set_name($name) {
$this->name = $name;
}
function get_name() {
return $this->name;
}
}$apple = new Fruit();
echo $apple->get_name();
echo “<br>”;
echo $banana->get_name();
?>
PHP – The __construct Function
A constructor allows you to initialize an object’s properties upon creation of the object.
If you create a __construct()
function, PHP will automatically call this function when you create an object from a class.
Example
<?php
class Fruit {
public $name;
public $color;function __construct($name) {
$this->name = $name;
}
function get_name() {
return $this->name;
}
}$apple = new Fruit(“Apple”);
echo $apple->get_name();
?>
PHP Iterables
PHP – What is an Iterable?
An iterable is any value which can be looped through with a foreach()
loop.
The iterable
pseudo-type was introduced in PHP 7.1, and it can be used as a data type for function arguments and function return values.
Example
Use an iterable function argument:
<?php
function printIterable(iterable $myIterable) {
foreach($myIterable as $item) {
echo $item;
}
}$arr = [“a”, “b”, “c”];
printIterable($arr);
?>
PHP Namespaces
Namespaces are qualifiers that solve two different problems:
- They allow for better organization by grouping classes that work together to perform a task
- They allow the same name to be used for more than one class
Namespaces are declared at the beginning of a file using the namespace
keyword:
Syntax
Declare a namespace called Html:
namespace Html;
<?php
echo “Hello World!”;
namespace Html;
…
?>